How to Upload Play by Play to Synergy
Here I am going to prove yous how to upload and play video using Django framework in Python programming. The uploaded video may or may not be played automatically just the uploaded video volition have controls, such equally, paly, interruption, full screen, mute, unmute, download, etc.
An upload grade is displayed to the end user for browsing and selecting a video file that will exist uploaded and displayed on the browser. I am using HTML5 tag to play the video. Make sure your browser supports HTML5 tag for playing video.
Prerequisites
Python: 3.9.v, Django: three.two.4
Project Setup
Yous generally create a Django project anywhere on your system and under your Django project you take one or more than applications.
Allow's say you accept created Django project directory calleddjangovideouploaddisplay and y'all have also created an app chosenvideouploaddisplay underdjangovideouploaddisplay. You lot may check the documentation for creating Django project and apps under project.
I presume yous have the required configurations for your videouploaddisplay in djangovideouploaddisplay/djangovideouploaddisplay/settings.py file underINSTALLED_APPS
section as below:
INSTALLED_APPS = [ ... 'videouploaddisplay.apps.VideouploaddisplayConfig', ]
Thevideouploaddisplay.apps.VideouploaddisplayConfig
is formed as a dotted notation from djangovideouploaddisplay / videouploaddisplay /apps.py, where you will find the class name equallyVideouploaddisplayConfig
that has proper name videouploaddisplay .
In your settings file – djangovideouploaddisplay/djangovideouploaddisplay/settings.py, defineMEDIA_ROOT
andMEDIA_URL
, for example:
MEDIA_ROOT = bone.path.bring together(BASE_DIR, '') MEDIA_URL = '/'
In the above configuration I am non specifying any directory for storing the video merely the video will be uploaded in the root directory of the project.

Template or View File
The template or view file is nothing but you lot write code on HTML file what you want to display on UI (User Interface). For this video file upload functionality I am going to put just one field that is file which volition exist used for browsing and selecting file.
The proper name of the below template file isupload-display-video.html and put it nethervideouploaddisplay/templates directory.
<!DOCTYPE html> <html> <caput> <title>Django - Video File Upload and Display</title> </head> <torso> <div style="width: 500px; margin: auto;"> <fieldset proper name="Video File Upload and Display"> {% if msg %} {% autoescape off %} {{ msg }} {% endautoescape %} {% endif %} <form method="post" activity="/" enctype="multipart/form-information"> {% csrf_token %} <dl> <p> <label>Browse and select a video file</label> <input blazon="file" proper noun="file" autocomplete="off" required> </p> </dl> <p> <input type="submit" value="Upload and Display"> </p> </course> </fieldset> {% if filename %} <div style="margin: 10px auto;"> <video autoplay="autoplay" controls="controls" preload="preload"> <source src="{{ MEDIA_URL }}/{{ filename }}" blazon="video/mp4"></source> </video> </div> {% endif %} </div> </body> </html>
In the above file user selects a video file and if file gets successfully uploaded into the root directory of the project folder so below the upload grade I am displaying the video that will starts playing automatically.
The another of import thing is yous demand to set CSRF token on the course using{% csrf_token %}
otherwise yous won't be able to upload file and you would get the following errors:
CSRF token is non fix CSRF Token missing or incorrect
You lot besides demand to ensure to cheque the CSRF token on your server side lawmaking and yous volition later when you lot get through the lawmaking inviews.py file.
Class
The DjangoForm
will map the fields to form on your template file. In the to a higher place template or view file I take kept simply one field calledfile
which is used to select a file. A view treatment this form volition receive data intoasking.FILES
.
request.FILES
will only incorporate data if the HTTP request method isPOST, at least i file is posted and the respective form has an attributeenctype="multipart/course-data"
, because dealing with forms that have FileField and ImageField fields are more circuitous.
The following code snippets are written intoforms.py file under directoryvideouploaddisplay.
from django import forms form UploadFileForm(forms.Form): file = forms.FileField()
Views
In this view I am going to show how to upload file into a server location. Yous will also see how file data is passed fromasking toForm
. Here also notice how I take used CSRF decorator to ensure the CSRF token.
from django.shortcuts import return from .forms import UploadFileForm from django.views.decorators.csrf import ensure_csrf_cookie @ensure_csrf_cookie def upload_display_video(request): if request.method == 'Mail': class = UploadFileForm(asking.POST, request.FILES) if course.is_valid(): file = asking.FILES['file'] #impress(file.name) handle_uploaded_file(file) render render(request, "upload-display-video.html", {'filename': file.name}) else: class = UploadFileForm() return render(asking, 'upload-display-video.html', {'course': grade}) def handle_uploaded_file(f): with open(f.name, 'wb+') as destination: for chunk in f.chunks(): destination.write(chunk)
Note that you lot have to pass request.FILES into theForm
's constructor to bind the file information into aForm
.
Looping overUploadedFile.chunks()
instead of usingread()
ensures that large files don't overwhelm your system's retentiveness.
Ideally the officehandle_uploaded_file()
should be put into mutual utility file, merely just for the sake of this instance I have kept into the aforementioned filevideouploaddisplay/views.py.
Earlier you save the file onto deejay the uploaded file data is stored under thetmp binder and the file name is generated something liketmpzfp312.upload. If the file size is up to 2.five MB then file is not saved nether thetmp binder and entire content is read from the memory and the reading becomes very fast.
URLs
At present you lot need to define the path or URL which will call the appropriate role (for this example,upload_display_video()
) on yourviews.py file. You need to create a fileurls.py undervideouploaddisplay binder with the following code snippets.
from django.urls import path from django.conf import settings from . import views urlpatterns = [ path('', views.upload_display_video, proper noun='upload_display_video'), ]
I want to upload and display video on root URL, so I did not mention any path on the URL.
Thepath()
function is passed four arguments, 2 required:route andview, and two optional:kwargs, andname.
road is a cord that contains a URL pattern.
view calls the specified view part with an HttpRequest object equally the beginning argument and whatsoever "captured" values from the route as keyword arguments.
kwargs are arbitrary keyword arguments tin be passed in a dictionary to the target view.
name – naming your URL lets y'all refer to it unambiguously from elsewhere in Django, especially from within templates.
Another important thing you need to do is to configure the URL into the project. This (videouploaddisplay) is an app and your projection does not know anything almost this URL. So add the following line intodjangovideouploaddisplay/djangovideouploaddisplay/urls.py file underurlpatterns = [...]
.
path('', include('videouploaddisplay.urls')),
You need to also include the MEDIA_URL which you lot defined before in settings.py file. The complete content for this djangovideouploaddisplay/djangovideouploaddisplay/urls.py file is given beneath:
#from django.contrib import admin from django.conf import settings from django.urls import path, include from django.conf.urls.static import static urlpatterns = [ #path('admin/', admin.site.urls), path('', include('videouploaddisplay.urls')), ] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
Deploying Application
Now I am ready to test the app I take built. Let'south burn upwardly the server from the command line usingmanage.py runserver
. The awarding volition run on default port8000
. If yous desire to change the default host/port of the server then you tin can read tutorial How to alter default host/port in Django.
Testing the Application
When y'all striking URLhttp://localhost:8000 on browser, your home page looks similar to the following prototype:

When yous upload a video your video will be started playing automatically. Hovering the video will display the controls for video.
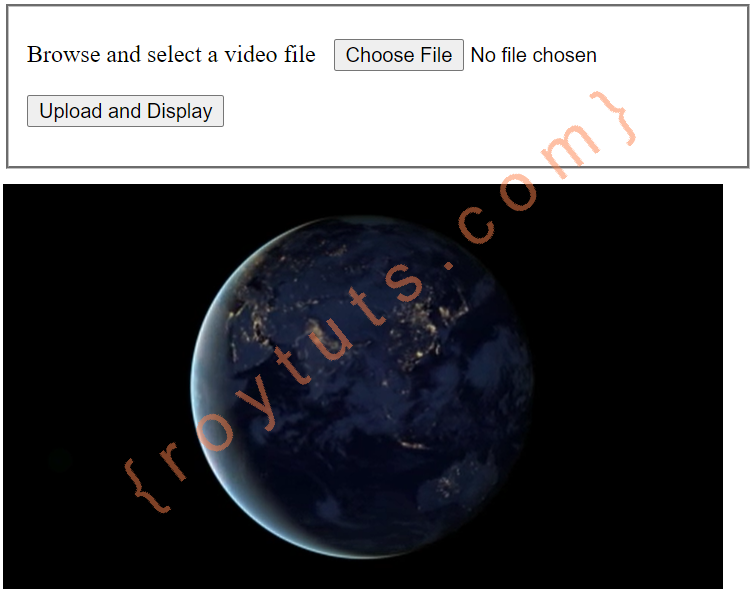
That'due south all about how to upload and play video using Django in Python.
Source Code
Download
Source: https://roytuts.com/upload-and-play-video-using-django/
0 Response to "How to Upload Play by Play to Synergy"
Post a Comment